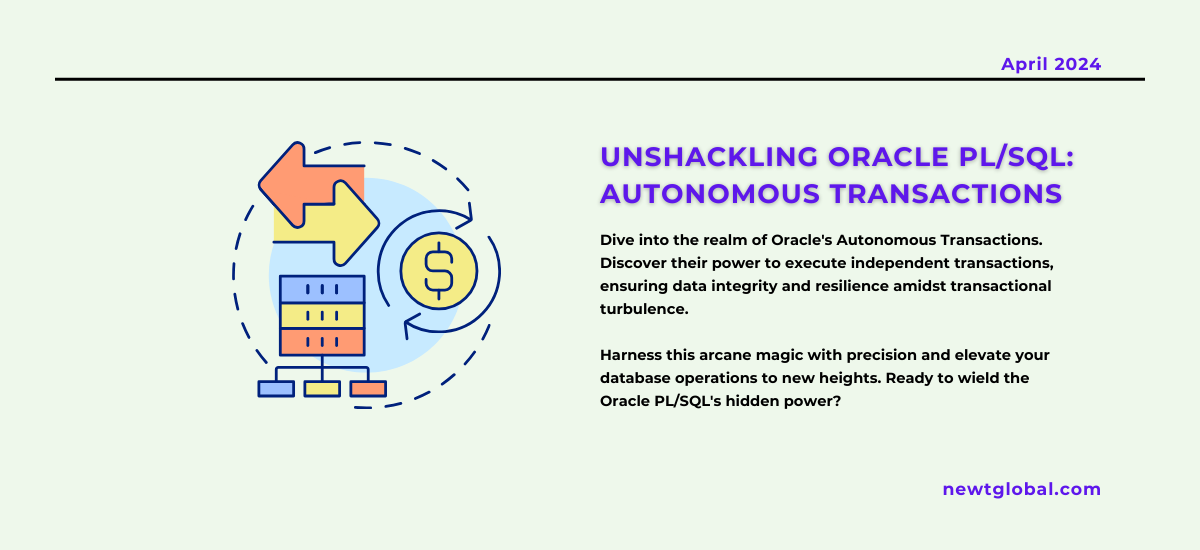
Oracle Database provides a robust environment for managing data and transactions. Among its many features, the Pragma Autonomous Transaction is a standout for its ability to execute SQL transactions in a separate transaction space. This article will delve into what Pragma Autonomous Transactions are, why they’re useful, and how to implement them with code examples.
Understanding Autonomous Transactions
In Oracle, a PRAGMA AUTONOMOUS_TRANSACTION; directive allows a transaction to be executed independently of its parent transaction. This means changes made by the autonomous transaction are committed or rolled back independently of the parent transaction’s fate. It’s particularly useful for writing to log tables, auditing, or performing operations that should not be rolled back even if the main transaction fails.
Why Use Autonomous Transactions?
Autonomous transactions have some key benefits:
-
- Isolation: They let you do operations separately, so any issues in the main transaction don’t affect them.
- Flexibility: You can let certain operations make changes without waiting for everything else to finish.
- Error Handling: They help catch and deal with errors smoothly while the main work keeps going.
Implementing Pragma Autonomous Transactions
To implement an autonomous transaction, you need to declare it inside a PL/SQL block, procedure, function, package, or trigger. Below are detailed examples to guide you through.
Basic Syntax
Before diving into complex scenarios, let’s understand the basic syntax:
CREATE OR REPLACE PROCEDURE procedure_name IS
PRAGMA AUTONOMOUS_TRANSACTION;
BEGIN
<executin_part>
[COMMIT|ROLLBACK]
END;
/
Example 1:
CREATE OR REPLACE PROCEDURE log_error(error_msg VARCHAR2) IS
PRAGMA AUTONOMOUS_TRANSACTION;
BEGIN
INSERT INTO error_log(message, log_date)
VALUES (error_msg, SYSDATE);
COMMIT; — Commit the autonomous transaction
END;
/
In the above example, the log_error procedure is declared with an autonomous transaction pragma. It inserts an error message into the error_log table along with the current timestamp and commits the transaction independently of the caller’s transaction.
Example 2:
CREATE OR REPLACE PROCEDURE lower_salary
(emp_id NUMBER, amount NUMBER)
AUTHID DEFINER AS
PRAGMA AUTONOMOUS_TRANSACTION;
BEGIN
UPDATE employees
SET salary = salary – amount
WHERE employee_id = emp_id;
COMMIT;
END lower_salary;
/
In this example, the lower_salary procedure is created with the AUTHID DEFINER clause, indicating that it executes with the privileges of its owner. The procedure decreases the salary of the employee identified by emp_id by the specified amount. The PRAGMA AUTONOMOUS_TRANSACTION pragma designates the procedure as an autonomous transaction, allowing it to commit its updates independently of the main transaction. After deducting the specified amount from the employee’s salary in the employees table, the procedure commits the changes to the database. This procedure can be useful in scenarios where salary adjustments need to be made separately from other transactions, ensuring data consistency and integrity.
The Conductor’s Beliefs: What to Do Best
-
- Use Your Powers Wisely: Only use this strong magic when you really need to, because having a lot of power means you have to be very careful.
- Finish What You Start: Always end your independent tasks by either making them official (commit) or cancelling them (rollback), so nothing gets left hanging.
- Have a Backup Plan: Make sure your independent tasks are well-protected against errors, so your data stays safe and everything works smoothly.
Conclusion
Discover the strength of Pragma Autonomous Transactions in Oracle to boost your database operations with unparalleled accuracy and dependability. With the guidance provided in this guide, you’re equipped to manage a series of separate transactions smoothly, each fulfilling its role perfectly in your overall data management plan.
Ready to leverage the power of autonomous transactions in your Oracle database management?
Take your expertise to the next level with Newt Global’s DMAP, a cutting-edge solution designed to streamline database operations and migration processes. Harness the potential of autonomous transactions alongside other advanced features to optimize your database performance. Learn more about DMAP and its capabilities at newtglobal.com and reach out to us at marketing@newtglobalcorp.com to embark on your journey towards mastering Oracle PL/SQL.
Newt Global DMAP is a world-class product enabling mass migration of Oracle DB to cloud-native PostgreSQL, faster, better, and cheaper.